Where to start on your Angular Project? if this is the question you have then this blog will be very helpful, In this blog we will explore Angular CLI to create/bootstrap angular project. We will use VSCode Node.js, NPM to setup an Angular project using Angular CLI and later clean up some of the code and add support for Jquery and Twitter Bootstrap in the project, also will showcase how to create a component in few seconds with Angular CLI.
It is suggested to read Angular CLI documentation at the official site:- https://cli.angular.io/.
Start your angular application using Angular CLI
Open your system and follow these point step by step to learn the approach:-
npm install -g @angular/cli
npm install -g @angular/cli@1.7.3
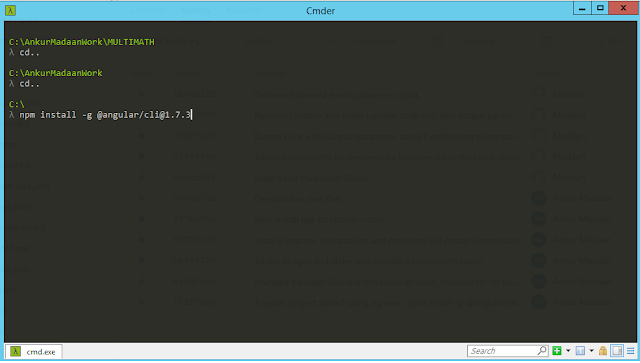
ng new {ProjectName}
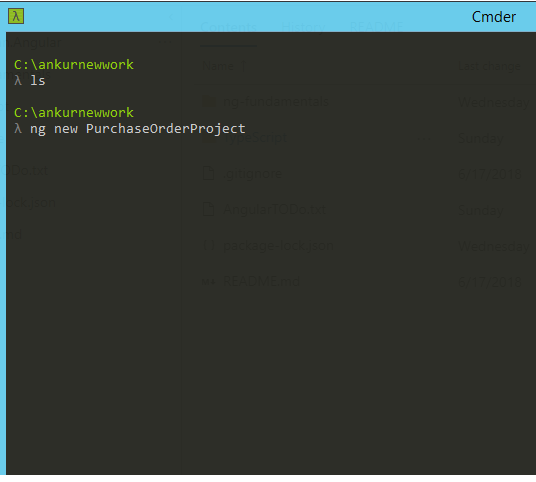
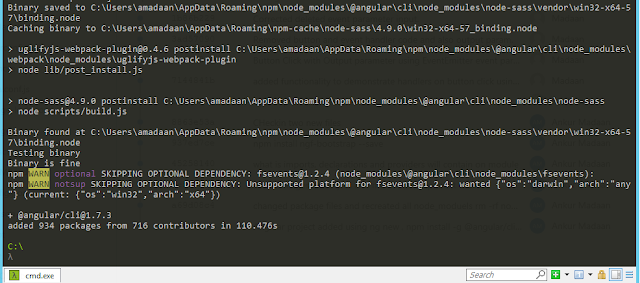
Note: Folder Name will be the same as of your ProjectName,
Next, lets open this folder in your fav Code editor.
I am using VSCode here, first thing to notice is package.json file
I am using VSCode here, first thing to notice is package.json file
- This package.json file is the one which tells npm which version of dependencies to install while generating the project. If you see closely you will notice ^ on top of version, it tells/dictates the page that atleast this version or greater than this version, we will stabilize this and keep most recent version here. so that in future if we migrate the code, there should be rights dependencies installed. If you hover on top of each setting it will tell you what is the current version available. You can leave the settings as it is if you want for always upgrading to latest ones.
- Next, remove node module folder to re install the dependencies files, open cmder and run this command to do it:-
rm -rf node_modules
- After this is done, we will reinstall dependencies by using npm install command:-
- Npm_modules will get back and all appropiate versions of the file gets installed.
- Now lets modify the port to run you application on angular-cli.json file, under scripts --start, write
ng serve --port 1200
- /This will run when we type npm start in command line and browse the location on 1200 port instead of default 4200 port. I do this just to control on which port i want to run my application:-
- type npm start in CMDER as shown above it will start your application, browse https://localhost:1200, it will open your Angular Application, like this:-
How to add external packages to the project?
Now lets see how to add other packages to your Angular Project, we will add twitter bootstrap and as it has jQuery Dependency we will add jquery too.
Too add Bootstrap, go to CMDER and run this command:-
npm install Bootstrap
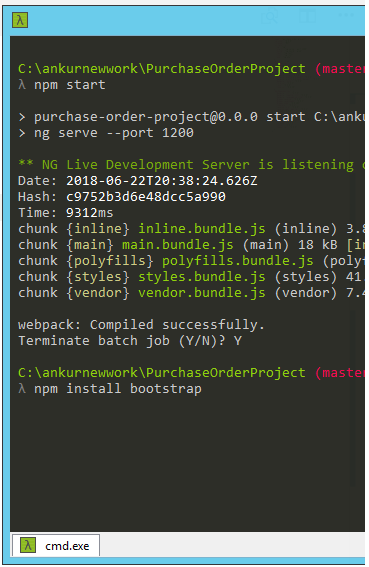
- It will install all dependency files of bootstrap to npm_modules folder and as well add a refference in Package.json file.
- Next add jQuery as bootstrap has dependency on it:-
npm install jQuery
And as recent bootstrap has dependency to Popper then install Popper.JS too as shown above.
- If you would like to install specific version of bootstrap and jquery then go to package.js folder and mention the specific version and then clear node_modules and npm install like we did earlier to get specific version of jquery and bootstrap.
- Next to use bootstrap and jquery in our current project we have to modify angular-cli.json file.
As shown in image above, go to Styles section an in the array include the location of bootstrap css file :- "../node_modules/boostrap/dist/css/bootstrap.min.css"
And to Scripts section in the value array put the Jquery file's path first and then the bootstrap's file's path. which is :-
jQuery :- "../node_modules/jquery/dist/jquery.min.js"
bootstrap:- "../node_modules/boostrap/dist/js/bootstrap.min.js"
These paths are in respect of index.html files as eventually on that file root module and components will get registers.
and if you do npm start in CMDER, you will see the bootstrap properties in action, just modify html file a little bit like add a container and row div.
Generate a new component using Angular CLI.
Its very easy to generate html template, component ts file and testing files using angular CLI, not only that it automatically registers the new component on the module. to do it you have to just type a simple command in your Command line editor:-
- We will create component for nav-bar at top of the page. For that just use this command to generate the component:-
ng generate component nav-bar
CLI will perform five steps first it will create html template for componenet, next the test file spec.ts, then the component itself and then the css file and finally update the main module to register component it has creeated, you can see these changes in your folder structure:-
You will see it has generated a new folder with the component name and respective files are automatically added to it.
CLI generally dictates this as best practice to create each component and its respective files under a single folder, you can nest multiple components under one component, and have them communicate with each other, thats not part of this blog though.
Lets delete the spec.ts file as its not needed as of now, i am not doing testing of anything, and just copy the code of Nav Bar from bootrstrap sample to the html template. And then add the on top of major app.component template to load the newly created nav component as shown here:-
Thats it, you should see nav bar added on your main page Will share more magic we can do with angular cli further in my blogs, please keep following.